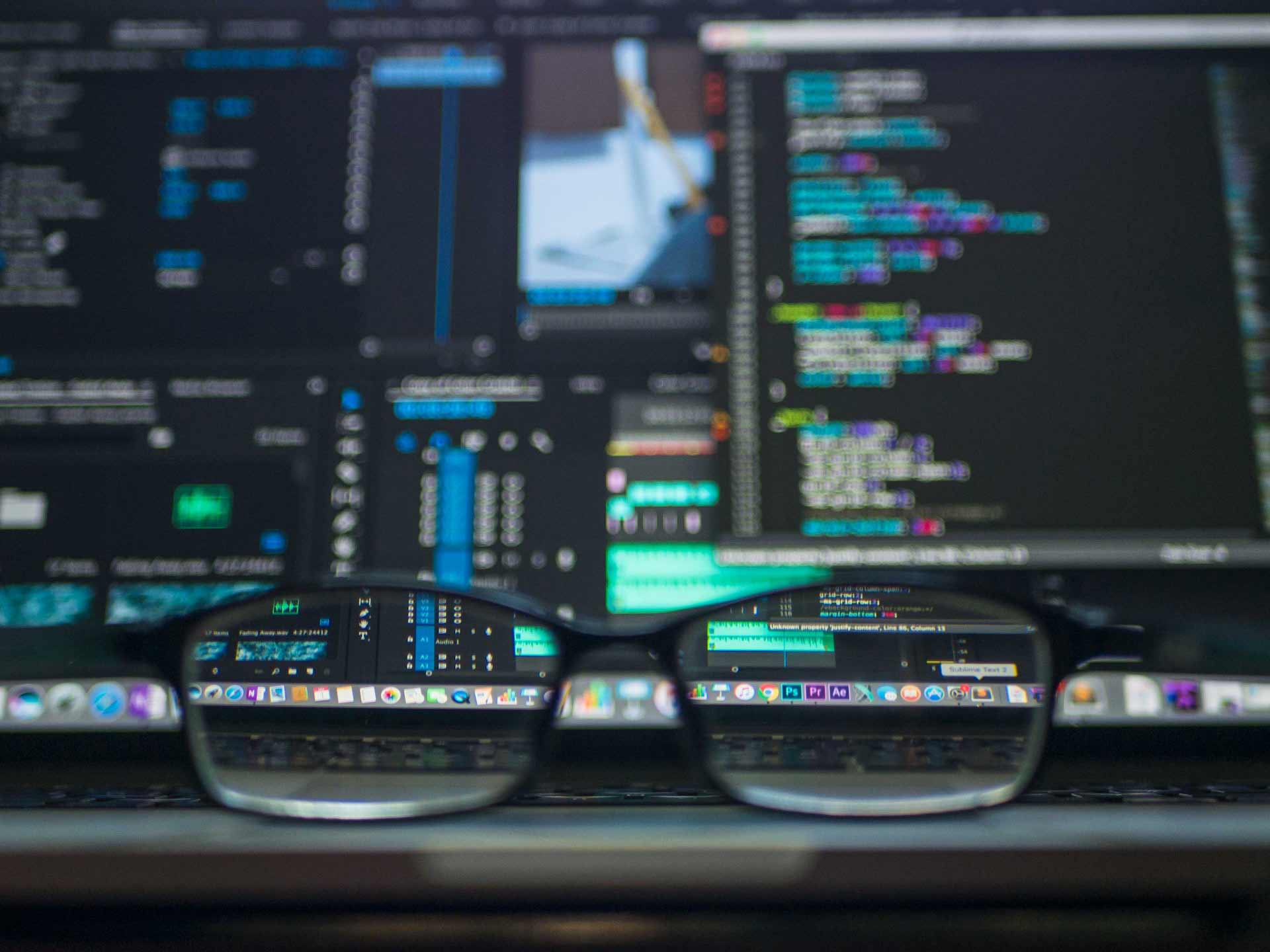
5 Reasons to learn Typescript
If you're a developer, you've likely heard of TypeScript. But what exactly is it and why should you invest your time in learning it? Here are five reasons why you should consider learning TypeScript:
.
1 - Easy implementation on existing JavaScript projects
You can start using TypeScript in new or refactored files and gradually adopt it in your existing project. This way you can take advantage of TypeScript's features without having to completely rewrite your existing codebase.
Whichever approach you choose, you can start by adding TypeScript to a small part of your project, and then gradually expand to other parts as you become more comfortable with the language. It's also important to note that you can use TypeScript and JavaScript files together in the same project, so you don't have to convert all of your JavaScript files to TypeScript at once.
It's worth noting that converting an existing project to TypeScript may require some work, but the benefits of using TypeScript will pay off in the long run with better code quality and maintainability.
Here is an example of a JavaScript code that could benefit from using TypeScript:
// JavaScript function to calculate the average of an array of numbers
function average(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
// Example usage:
let grades = [90, 85, 78, 92, 88];
console.log(average(grades));
.
The above code is a simple example of a JavaScript function that calculates the average of an array of numbers. However, there are a few issues with this code:
- There is no way to know if the argument passed to the function is an array of numbers, or any other type of array.
- There is no way to know the return type of the function.
TypeScript can help to solve these issues by providing type annotations for function arguments and return values. Here's how the same code could be written in TypeScript:
// TypeScript function to calculate the average of an array of numbers
function average(numbers: number[]): number {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
// Example usage:
let grades: number[] = [90, 85, 78, 92, 88];
console.log(average(grades));
.
With TypeScript, the function's argument type is explicitly defined as an array of numbers, this makes it clear that the function expects an array of numbers as an input. It also defines that the function will return a number. This can prevent errors and make the code more maintainable. Also, TypeScript will check the types of the argument passed to the function, so if you pass an argument that is not an array of numbers to the average function, you will get a compile-time error, instead of a run-time error.
It's worth noting that this is just a simple example, and in a real-world project, you may need to use more advanced features of TypeScript, such as interfaces, classes, and modules, to structure your code and manage dependencies. But, even with a simple example like this, you can see how TypeScript can help to improve the quality and maintainability of your code.
.
2 - TypeScript provides static type checking
When you write JavaScript code, it's easy to make mistakes, such as passing the wrong type of data to a function or accessing an object property that doesn't exist. TypeScript's static type checking helps you catch these errors before you run your code, making it easier to write more robust and maintainable code.
function add(a: number, b: number): number {
return a + b;
}
let result = add(1, 2); // This is fine, result is a number
result = add("1", "2"); // This will cause an error, because the arguments are not numbers
.
In this example, the function "add" is defined with type annotations for its arguments and return value, which means that TypeScript will check that the arguments passed to the function are of the correct type (numbers) and that the return value is also a number. If you try to call the function with the wrong types of arguments, TypeScript will give you an error.
.
3 - TypeScript has better support for large and complex projects
As your projects grow in size and complexity, it becomes harder to keep track of all the different parts and how they interact. TypeScript's static type checking and its advanced features, such as interfaces and classes, make it easier to structure your code and manage dependencies.
interface Point {
x: number;
y: number;
}
class Line {
constructor(public start: Point, public end: Point) {}
length(): number {
let dx = this.end.x - this.start.x;
let dy = this.end.y - this.start.y;
return Math.sqrt(dx * dx + dy * dy);
}
}
.
In this example, we have an interface "Point" that defines the shape of an object with x and y properties. We also have a class "Line" that has a constructor that takes two points and it also has a method called length which will calculate the distance. This makes it easy to understand how the different parts of the code are related and how they interact, which can be especially helpful in large and complex projects with many different components.
.
4 - TypeScript is gaining popularity
As more and more companies adopt TypeScript, the demand for developers who know it is increasing. Learning TypeScript can help you stand out from other developers and make you more attractive to potential employers.
Here are a few examples of data that demonstrate this trend:
- According to the Stack Overflow 2020 Developer Survey, TypeScript is the most loved programming language among developers, with 74.5% of respondents saying that they want to continue working with it.
- According to GitHub's State of the Octoverse 2020 report, TypeScript is the fastest-growing language in terms of pull requests and contributors, with a growth rate of 190% over the past year.
- According to Google Trends, the interest in TypeScript has been steadily increasing over the past few years, and is currently at an all-time high.
- According to the RedMonk Programming Language Rankings, TypeScript has moved up the rankings from the 19th most popular language in 2019 to the 14th most popular in 2020.
- According to Indeed.com, job postings that list TypeScript as a required skill have increased by 150% since 2016.
These statistics demonstrate that TypeScript is becoming increasingly popular among developers, and that its usage and popularity are on the rise. Companies like Asana, Airbnb, Asos, AWS, Azure, Babel, Baidu, Basecamp, Behance, Codeship, GitHub, InVision, Microsoft, Nrwl, Slack, and many more are using TypeScript for their development.
It's worth noting that this data is subject to change over time, but it gives a good idea of how TypeScript's popularity has grown. It's also worth noting that even though TypeScript is gaining popularity it's not a replacement of JavaScript, but rather a complement to it.
.
5 - TypeScript is open-source and actively developed
TypeScript is developed and maintained by Microsoft, and has a growing and active community of contributors. This means that you can be sure that the language will continue to evolve and improve, and that you'll have a wealth of resources and support to help you learn and use it.
There are many resources available to help you learn TypeScript. Here are a few options that you can consider:
- Here on Telmo Academy, we have plenty of courses that will help you learn Typescript, and how you can use it in real projects.
- The TypeScript official website provides a wealth of resources for learning the language, including a comprehensive handbook, tutorials, and a playground where you can experiment with TypeScript code.
- The TypeScript documentation (typescriptlang) is another great resource for learning the language, it covers all the concepts of TypeScript and it's easy to follow.
In conclusion, TypeScript is a powerful language that can help you write better and more maintainable code. Its static type checking, support for large and complex projects, and growing popularity make it a valuable skill for any developer to learn. If you're not already using TypeScript, consider giving it a try – you may be surprised by how much it can improve your workflow and the quality of your code.
Written by Telmo Sampaio | Published - 21 January 2023