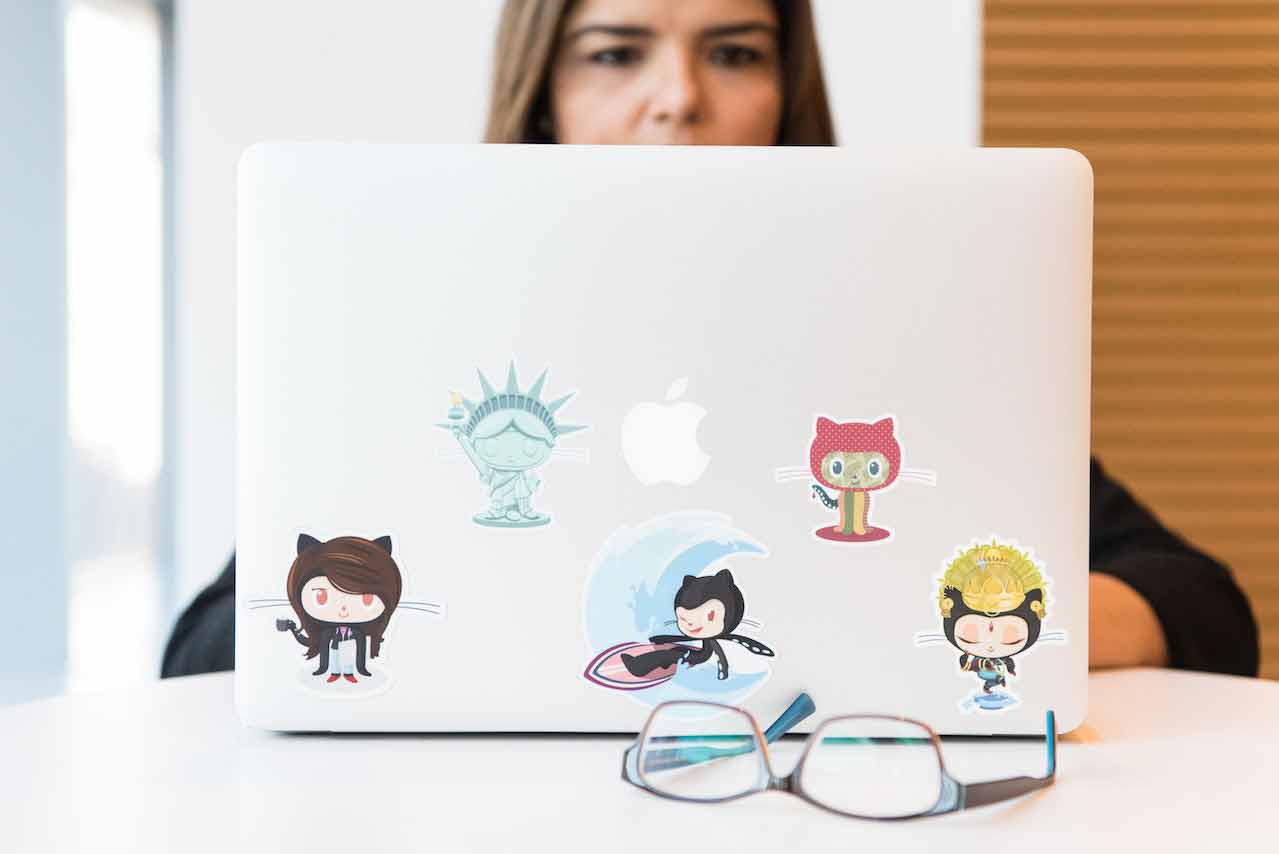
How to save your uncommited code with Git Stash
Have you ever been in the middle of a bug fix, or working in a certain feature, and suddenly you need to change to another branch, but you don't really want to commit your code because it's not finished.
.
Well that's a great example where you could use Git Stash.
.
Git stashes allow you to temporarily save changes in your working directory without committing them to the repository. This can be useful if you need to switch to a different branch or work on a different task, but don't want to commit your changes yet.
.
Here are some examples of how to work with Git stashes:
.
1 - Create a stash:
To create a stash, run the following command:
git stash save "your message"
This will save your changes in a new stash with the message "your message".
.
2 - View your stashes:
To view a list of your stashes, run the following command:
git stash list
This will show you all of your stashes, with the most recent one at the top.
.
3 - Apply a stash:
To apply a stash, run the following command:
git stash apply stash@{n}
Replace n with the number of the stash you want to apply. If you don't specify a stash number, Git will apply the most recent stash.
.
4 - Apply and drop a stash:
To apply a stash and remove it from the stash list, run the following command:
git stash pop stash@{n}
Replace n with the number of the stash you want to apply and drop. If you don't specify a stash number, Git will pop the most recent stash.
.
5 - Apply only specific files from a stash:
If you only want to apply specific files from a stash, you can use the git stash apply command with the --index flag and specify the files you want to apply. For example:
git stash apply --index stash@{n} path/to/file1 path/to/file2
.
6 - Create a new branch from a stash:
To create a new branch from a stash, run the following command:
git stash branch new-branch-name stash@{n}
Replace new-branch-name with the name of the new branch you want to create, and n with the number of the stash you want to apply to the new branch.
.
7 - Drop a stash:
To remove a stash from the stash list without applying it, run the following command:
git stash drop stash@{n}
Replace n with the number of the stash you want to drop.
.
8 - Clear all stashes:
To remove all stashes from the stash list, run the following command:
git stash clear
.
Hope you guys enjoyed this tutorial